CC Game Programming News
Your source for news, events, contests, and resources for Game Programmers at Champlain College.
Monday, October 24, 2011
Microsoft on Silverlight and XNA
On Thursday the 27th of October Microsoft will be on campus all day hosting discussions on Silverlight, XNA and developing on Windows Phone 7. This will be from 9:00am to 5:00pm and registration is required as well as printed tickets. This will be taking place in the Hauke Family Center and will be a great opportunity to learn more about mobile development and chance to meet the folks at Microsoft.
Students can register here: http://wp7mangoburlington.eventbrite.com/
Labels:
Microsoft,
register,
Silverlight,
WP7,
XNA
Friday, October 14, 2011
XNA Networking Events, In a Nutshell
In my explorations of Microsoft's XNA and its networking features, it quickly became apparent that utilizing events makes life much easier. Managing game states in a networked game can be a pain. The most basic states are when you are picking a session to join, then when you are in a lobby of other players waiting to play the game, finally to actually playing the game. Although it would be possible to switch between these states without using some of XNA's baked in networking events, it results in much more complex code that is more likely to break and generally cause headaches. So here are some tips.
When you have successfully joined or created a NetworkSession, the first thing you should do in your lobby is hook the following events:
So, how do you hook these events? Its simple, lets take GameStarted for example:
When you have successfully joined or created a NetworkSession, the first thing you should do in your lobby is hook the following events:
NetworkSession.GamerJoined
NetworkSession.GamerLeft
NetworkSession.SessionEnded
NetworkSession.GameStarted
NetworkSession.StartGame()is called somewhere in your code. The advantage of using the start game function in your code is that the GameStarted event will be fired to each player. Letting you centralize any initialize anything you need to before the game starts.
NetworkSession.GameEnded
NetworkSession.EndGame(). Once again, allows you to transition the player to another state. The most likely scenario is changing to the lobby screen when this happens.
So, how do you hook these events? Its simple, lets take GameStarted for example:
// Assuming your NetworkSession is stored in CurrentSessionGot it? Good. Now you are ready to tackle the actual game logic! That will have to wait until another time though.
private void HookSessionEvents()
{
//hook all your events here, just using this one as an example
CurrentSession.GameStarted += CurrentSession_GameStartedHandler;
}
// change screen
private void gameStarted(object sender, GameStartedEventArgs e)
{
// Reset everything when we are starting a new game.
NetworkSession session = (NetworkSession)sender;
for (int i = 0; i < session.AllGamers.Count; i++)
{
GamerObject gOjbect = session.AllGamers[i].Tag as GamerObject;
gObject.Reset();
}
// show message on the screen for 3 seconds
ScreenManager.AddMessage("START THE GAME FOOL!", 3000);
// switch to your game screen / state here
}
Thursday, October 13, 2011
Remembering Dennis Ritchie
Although very familiar with Bjarne Stroustrup as the inventor of C++, I am sad to say that, until this week, I did not have the name of Dennis Ritchie and his achievements committed to memory. However, his achievements have been some of the greatest contributions to computer science and development which the world continues to feel today. At this point if you, like me, were once unfamiliar with Dennis Ritchie, let's learn a little bit more about this man and what he did for us all.
Dennis MacAlistair Ritchie (username: dmr) was born September 8, 1941 in Bronxville NY. He attended Harvard University and left with degrees in physics and applied mathematics. In 1968 he earned his Ph.D. He started work at Bell Labs in 1967 in the Computing Sciences Research Center where he worked on the famous project Multics. When Multics was cancelled, the team then decided to redo the project on a smaller scale stating
What we wanted to preserve was not just a good environment in which to do programming, but a system around which a fellowship could form. We knew from experience that the essence of communal computing, as supplied by remote-access, time-shared machines, is not just to type programs into a terminal instead of a keypunch, but to encourage close communication.This created what is today known as UNIX.
During the development of UNIX, around 1972, Brian Kernighan and Dennis Ritchie wanted to port the operating system to a PDP-11. However, B was unable to take advantage of some of the PDP-11's features, notably byte addressability, so they decided to develop C. In 1973, C had become robust enough that UNIX's kernel was mostly written in C which gave rise to the first portable operating system.
In 1978 Brian Kernighan and Dennis Ritchie published the first book on C called The C Programming Language from which we get the genesis of the "Hello World" code sample. Consequently, almost all of today's programming languages can be traced back to or have been influenced by C. Today, almost all computer operating systems are written in C or some derivative of the language. Without the invention of the C computer languages, development may have ended up a lot differently.
Sadly, this weekend, Dennis Ritchie left us. It was first reported by Rob Pike via Google+ that the computer scientist had passed due to a long battle with an unspecified illness. Although he may not be as well known as other pop celebrities who have died in the past year, there is no doubt in my mind that this man's achievement has influenced and changed our world more than any entertainer could ever warrant. So on that note, we say good bye to a great and brilliant man.
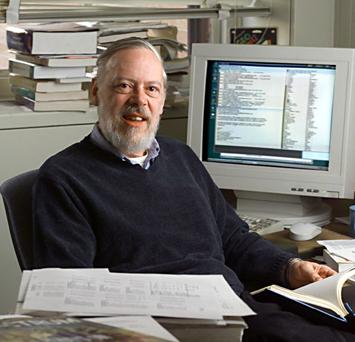
RIP
Dennis MacAlistair Ritchie
1941-2011
Dennis MacAlistair Ritchie
1941-2011
Friday, October 7, 2011
Game Development Summer Internship
It seems that Microsoft has taken notice of the game development students here at Champlain and are very interested in hiring students or recent graduates for Game Programming, among other fields, for Summer 2012. Internships will be paid great money with possible housing and transportation. But anyone interested in a position has to act fast, Microsoft's hiring for Summer 2012 ends in November so get your resumes primped and contact Mark Zammuto posthaste!
source: http://pilechamplain.blogspot.com/2011/10/oppertunities-and-information.html
source: http://pilechamplain.blogspot.com/2011/10/oppertunities-and-information.html
Labels:
game programmers,
internship,
job,
Microsoft,
new grad
Saturday, October 1, 2011
Using AppHub and MSDN as Resources for XNA Game Development
The internet is one of the greatest resources for learning how to code. Do a quick Google search for a specific topic and you'll most likely find five or more answers on StackOverflow, a dozen blogs that walk you through with code and explanations, and most certainly a forum post with plain text code pasted into the question with no answers following it. It's truly amazing how much time people spend trying to help others. However, the one big pitfall is the sheer amount of resources you can find on the internet. It is overwhelming.
For example, when I do a Google search for "pixel shaders in XNA," I get About 141,000 results (0.29 seconds). Wow! No purchasing any book, no sending an email to a professor asking for help, etc. I can just click on one of the ten links on the first page of Google and pray it's what I need. If it's not, then try another one. The big problem is that code becomes outdated very quickly. The weight of a blog post or video in search engines wasn't weighted on date published until recently. That's also assuming there is a more recent version available somewhere on the web as well.
If someone wrote a blog post on pixel shaders in XNA 3.x, what you're going to get out of that blog post is much less than a post on XNA 4.0 (assuming that's what you're using). My point is, it's overwhelming when you Google for solutions, especially when it's programming related. It might be outdated, it might not fit into your project, or the sample code might be flatout wrong.
My suggestion to fellow coders and specifically people working with XNA is to use the sources that the creator of that framework or tool have published. Whether it's an API, wiki, or just code examples - the chances are much higher that it will work, follow best practices, and be up to date.
When XNA 4.0 was released, Microsoft went back and updated all of their old 3.x projects with 4.0 solutions and code. This means that if you're using XNA 4.0, anything on the Microsoft website will be of use and work with your current configuration. So now things are boiled down to the resources from the people who put whatever you're using out there. But it's still overwhelming!
In Microsoft's AppHub content catalog, there are 185 results for their education section. Where do you even start? There's sections for Windows Phone 7 development, XNA, Windows PC, and all this new stuff about Mangos?! Well there is hope for you yet - deep within the menus, content, and tutorials there are some awesome resources for XNA developers.
If you're new to XNA, I would reccomend checking out their Game Development Tutorial. It will walk you through starting a game from scratch to having animations, bullets, and enemies. There are even videos, source code zips, and in-depth explanations. These are great for getting the hang of XNA and learning the basics.
If you're not so new to XNA, I would reccomend checking out the XNA Game Studio 4.0 library. It covers a ton of topics, ranging from packaging and distributing your game to the content pipeline already set up in XNA.
If you're looking for something specific, then search the content catalog or narrow your results down based upon the type of content, dev area, or platform.
If you're looking for the nitty-gritty of XNA, the Framework Class Library, then this will be a huge help. Take your time, read through what interests you, and search for the things you can't directly find.
While some people understand how to go through an API, others may be scared to go through such a large amount of information. If you're someone who is scared, then take your time and learn to love it. Well-documented APIs are insanely helpful to programmers.
Hopefully this was a help to get started with XNA, finding help, and learning. While there are tons of great resources out on other places in the web, MSDN and AppHub aren't bad starting places.
Good luck, and feel free to ask any questions!
Labels:
programming,
XNA
Friday, September 30, 2011
Lab Hours
Skiff 102 Lab | ||||
---|---|---|---|---|
Monday | Tuesday | Wednesday | Thursday | Friday |
9am-5:30pm | 11am-2pm | 9am-2pm | 9am-2pm | 3:15pm-8:15pm |
3:30pm-5:30pm | 3:30pm-5:30pm | 3:30pm-5:30pm | 3:15pm-8:15pm |
West Hall Lab | ||||
---|---|---|---|---|
Monday | Tuesday | Wednesday | Thursday | Friday |
9:30am-8pm | 9am-3pm | 9:30am-1:30pm | 10am-8pm | 9am-5:30pm |
3:30pm-8pm | 2pm-8pm |
Library G08 | ||||
---|---|---|---|---|
Monday | Tuesday | Wednesday | Thursday | Friday |
9am-2pm | 11am-2pm | 9am-11am | 10am-2pm | 12:30pm-4:30pm(until 11/10/11) |
4pm-5:30pm | 3:30pm-5:30pm | 1:30pm-5:30pm |
Foster 104 | ||||||
---|---|---|---|---|---|---|
Monday | Tuesday | Wednesday | Thursday | Friday | Saturday | Sunday |
8pm-11pm | 8pm-11pm | 8pm-11pm | 3pm-6pm | 3pm-7pm | 10am-6pm | 11am-6pm |
8pm-11pm |
Hauke 008 | ||||||
---|---|---|---|---|---|---|
Monday | Tuesday | Wednesday | Thursday | Friday | Saturday | Sunday |
Flash Lab | 5pm-9pm | 7pm-11pm | 6pm-11pm | 11am-11pm | 2pm-6pm | |
8pm-12am | 8pm-11pm | 7pm-11pm |
Hauke 007 | ||||||
---|---|---|---|---|---|---|
Monday | Tuesday | Wednesday | Thursday | Friday | Saturday | Sunday |
2pm-6pm | 9pm-12am | 7pm-11pm | 5pm-11pm | 5pm-8pm | 1pm-6pm | 1pm-6pm |
Thursday, September 29, 2011
New Site for Game Programmer News
This is a new site designed to share information for student studying Game Programming at Champlain College.
The site is maintained with the help of Game Programming Professor, John Pile and work study students. If you'd like to contribute, John know.
Subscribe to:
Posts (Atom)